Understanding Depth-First Search (DFS) is essential for anyone looking to delve into the world of algorithms and data structures. Among the various approaches to implement DFS, utilizing a stack is one of the most efficient methods. A stack-based DFS offers a unique perspective on exploring graphs and tree structures, making it an indispensable tool for programmers and computer scientists alike. In this article, we will explore how DFS using stack works, its advantages, implementation details, and practical applications. Whether you are a beginner eager to learn about DFS or an experienced developer seeking to refine your skills, this guide will provide valuable insights.
Depth-First Search is a fundamental algorithm used to traverse or search through data structures like trees and graphs. The stack-based approach allows for backtracking, which is crucial when navigating complex structures. By using a stack, we can keep track of the nodes visited and efficiently manage the order of exploration, ensuring that we explore as far as possible along each branch before backtracking.
Throughout this article, we will answer key questions related to DFS using stack, including its implementation, advantages over recursive approaches, and how it can be applied in real-world scenarios. By the end of this guide, you will have a solid understanding of DFS using stack and how to implement it in your programming projects.
What is DFS Using Stack?
DFS using stack is a method of implementing the Depth-First Search algorithm through the use of a stack data structure. Unlike the recursive approach, which uses the call stack implicitly, this method allows for explicit control over the nodes being visited.
How Does DFS Using Stack Work?
The stack-based DFS algorithm works by pushing the root node onto the stack, marking it as visited. Then, we repeatedly pop nodes from the stack, exploring their unvisited neighbors and pushing them onto the stack. This continues until all nodes have been visited or the stack is empty.
Why Choose Stack Over Recursion for DFS?
There are several reasons to prefer using a stack for DFS over a recursive approach:
- Control: Using a stack gives you explicit control over the traversal order.
- Memory Efficiency: It can be more memory-efficient than recursion, especially for deep trees.
- Flexibility: A stack can be easily modified or replaced with another data structure if needed.
How to Implement DFS Using Stack in Code?
Implementing DFS using stack can be done in various programming languages. Below is an example implementation in Python:
def dfs_using_stack(graph, start): visited = set() stack = [start] while stack: vertex = stack.pop() if vertex not in visited: visited.add(vertex) stack.extend(neighbor for neighbor in graph[vertex] if neighbor not in visited) return visited
What Are the Applications of DFS Using Stack?
DFS using stack has numerous applications in various fields. Some of the most common applications include:
- Pathfinding algorithms in AI.
- Solving puzzles like mazes and Sudoku.
- Topological sorting in directed graphs.
- Finding connected components in a graph.
What Are the Advantages of DFS Using Stack?
DFS using stack offers several advantages:
- Iterative Approach: It eliminates the risk of stack overflow, which can occur with deep recursion.
- Easy Backtracking: The stack facilitates easy backtracking to explore different branches.
- Clear Implementation: The algorithm can be clearly articulated in code without hidden complexities.
How to Optimize DFS Using Stack?
Optimizing DFS using stack can enhance performance and reduce memory usage. Here are some optimization techniques:
- Use an iterative deepening approach: This combines the benefits of depth-first and breadth-first search.
- Limit depth: Set a maximum depth to prevent excessive memory use.
- Use a visited set: Keep track of visited nodes to prevent cycles in graphs.
What Challenges Can Arise with DFS Using Stack?
While DFS using stack is powerful, there are challenges to consider:
- Handling cycles: Cycles in graphs can lead to infinite loops if not managed properly.
- Memory constraints: Large graphs can consume significant memory, requiring efficient data handling.
- Complexity: Understanding and implementing the algorithm can be complex for beginners.
In conclusion, DFS using stack is an essential algorithm that offers a robust way to traverse and explore data structures. Its advantages, such as explicit control, memory efficiency, and flexibility, make it a preferred choice for many applications. By mastering DFS using stack, you can enhance your problem-solving skills and develop more efficient algorithms for various tasks.
Article Recommendations
- Macd For Ym
- Quality Metal Detectors
- Freddie Prinze Jr Jessica Biel
- Hdfs Copy To Local
- Ixora Maui Yellow
- Drinking Ambien
- Large Living Room Cabinet
- Vogue October 2003
- Robin Sharma Wife
- Ap Precalculus Unit 3 Review


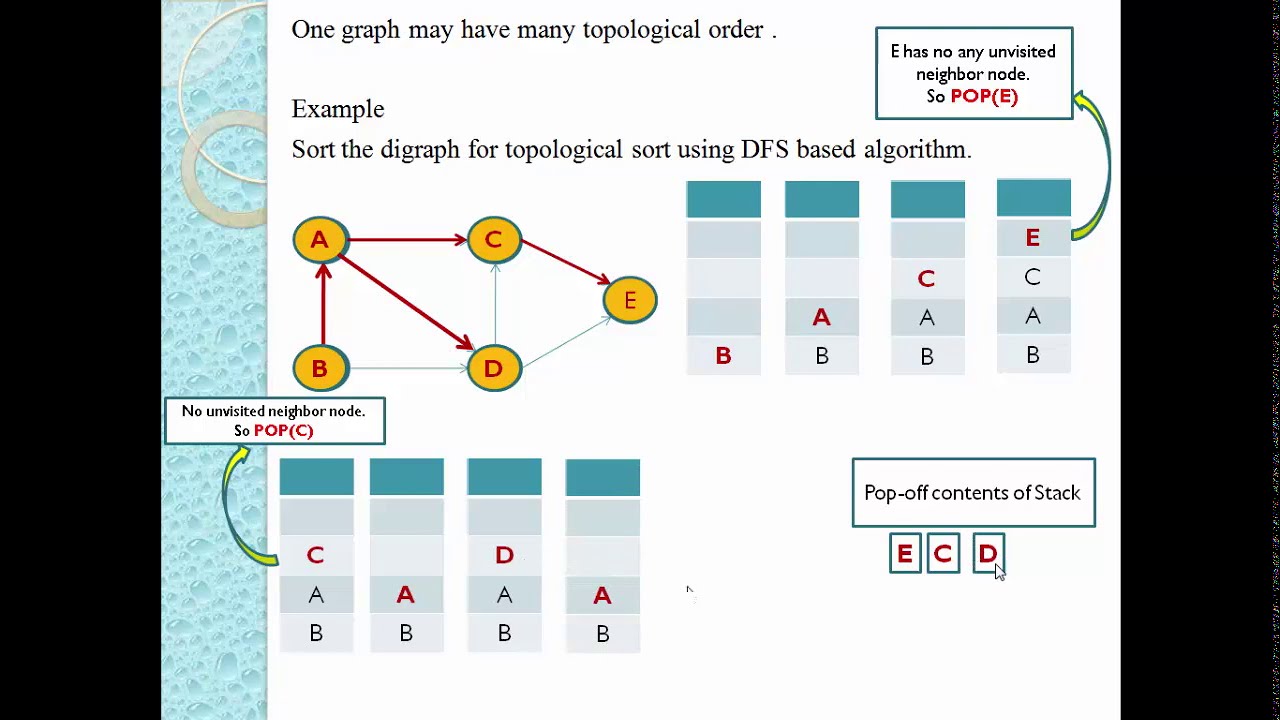