C# is a versatile and powerful programming language, widely used in various applications, from web development to game design. One of the most common constructs in C# is the foreach loop, which simplifies the process of iterating through collections. However, developers often encounter scenarios where they need to exit the loop prematurely. This is where the "C# foreach break" statement comes into play. In this article, we will explore the nuances of using the break statement within a foreach loop, enhancing your understanding of C# control flow.
As you delve into this guide, you will uncover the intricacies of the foreach loop, the purpose of the break statement, and practical examples that illustrate their combined usage. Whether you are a novice programmer looking to grasp the fundamentals or an experienced developer seeking to refine your skills, this article will equip you with the knowledge needed to effectively utilize the "C# foreach break" statement in your code. Let's embark on this journey to master control flow in C#.
Understanding the "C# foreach break" is essential for writing efficient and clean code. It allows you to manage the flow of your programs, ensuring that your loops behave as intended. By the end of this article, you will have a solid grasp of how to implement break statements effectively within foreach loops, enhancing both the performance and readability of your code.
What is a Foreach Loop in C#?
The foreach loop is a control flow statement that enables you to iterate through collections, such as arrays, lists, and dictionaries, without the need for an explicit index. This makes it a favorite among developers for its simplicity and readability. In a foreach loop, you define a variable that takes on the value of each element in the collection, allowing you to perform operations on it seamlessly.
How Does the Foreach Loop Work?
To understand the mechanics of the foreach loop, consider the following syntax:
foreach (var item in collection) { }
Here, item
represents the current element being processed, and collection
is the collection you are iterating over. The loop will continue to execute until all elements have been processed, making it an ideal choice for traversing collections.
What is the Purpose of the Break Statement?
The break statement is a control flow statement used to exit loops prematurely. When a break statement is encountered within a loop, the loop terminates immediately, and control is transferred to the statement following the loop. This is particularly useful when you need to stop processing based on certain conditions.
How to Use C# Foreach Break?
Using the break statement within a foreach loop is straightforward. You simply include the break statement within the loop's body, typically inside an if statement that defines the condition under which the loop should terminate. Here is an example:
foreach (var number in numbers) { if (number > 10) { break; // Exit the loop if the number is greater than 10 } Console.WriteLine(number); }
In this example, the loop iterates through a collection of numbers. If a number greater than 10 is encountered, the loop will break, preventing any further iterations.
When Should You Use C# Foreach Break?
There are several scenarios where using the break statement in a foreach loop can be beneficial:
- When you want to stop processing once a specific condition is met.
- To improve performance by avoiding unnecessary iterations.
- When you are searching for an item and want to exit once it's found.
What Are the Best Practices for C# Foreach Break?
To ensure that your usage of the break statement is effective and maintains code readability, consider the following best practices:
- Keep your conditions clear and concise.
- Avoid deeply nested loops that may complicate logic.
- Document your code to explain why the loop is being exited.
Can You Use Break with Other Loop Types?
Absolutely! The break statement is not limited to foreach loops. You can use it with other loop constructs in C#, including:
- for loops
- while loops
- do-while loops
In each case, the break statement will function similarly, allowing you to exit the loop based on specified conditions.
What Are Some Common Mistakes When Using C# Foreach Break?
While using the break statement can simplify your code, some common mistakes to avoid include:
- Forgetting to include a condition, which can lead to unintended behavior.
- Using break statements in nested loops without clarifying which loop is being exited.
- Overusing break statements, which can make code harder to follow.
Conclusion: Mastering C# Foreach Break
Understanding the "C# foreach break" statement is crucial for any developer looking to write efficient and maintainable code. By mastering the foreach loop and the break statement, you'll be equipped to control the flow of your applications effectively, creating cleaner and more efficient programs. Remember to use break statements judiciously, and always strive for clarity in your code.
With this knowledge in hand, you're now ready to incorporate the "C# foreach break" into your programming toolkit, enhancing both your coding skills and the performance of your applications.
Article Recommendations
- Elasticized Belt
- Bi Fold Exterior Patio Doors
- Drinking Ambien
- Luisa Baratto
- Ui For Apache Kafka Value Filter
- Elements Compounds And Mixtures Answer Key
- How To Use Rabbitfx
- Horny In Sign Language
- Free Attractions In Niagara Falls
- Glycemic Index Tomato Sauce

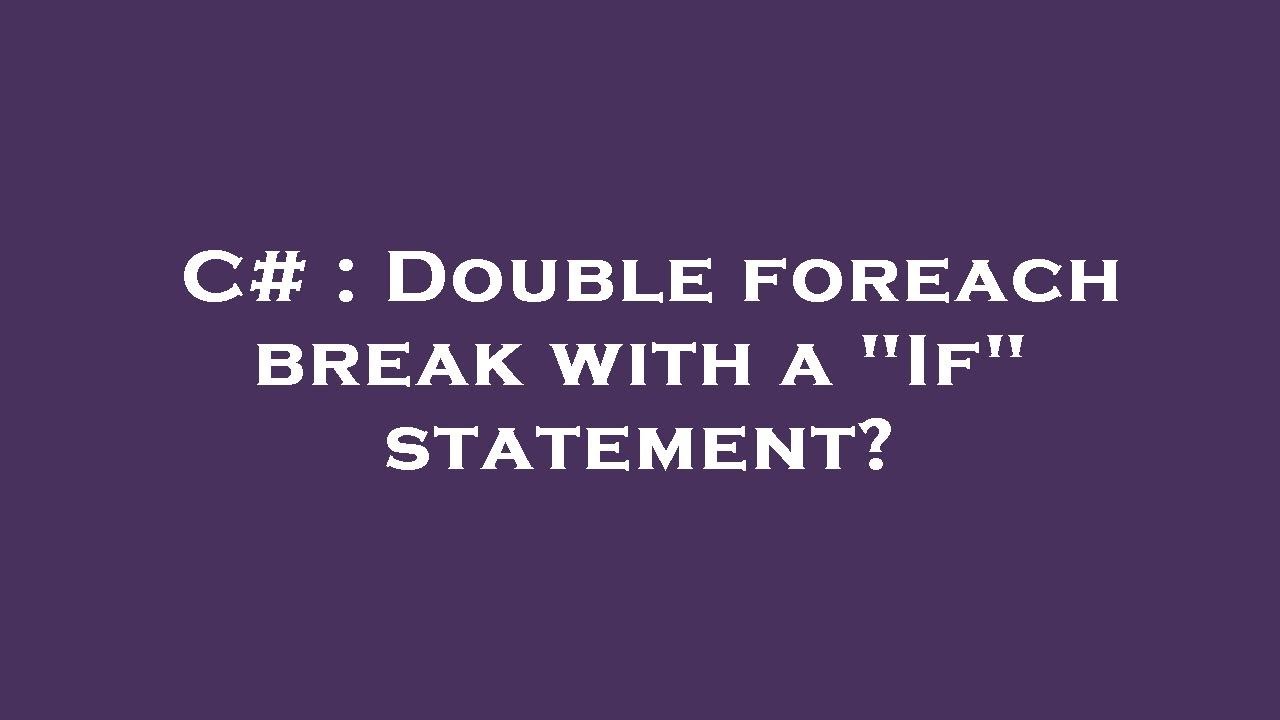
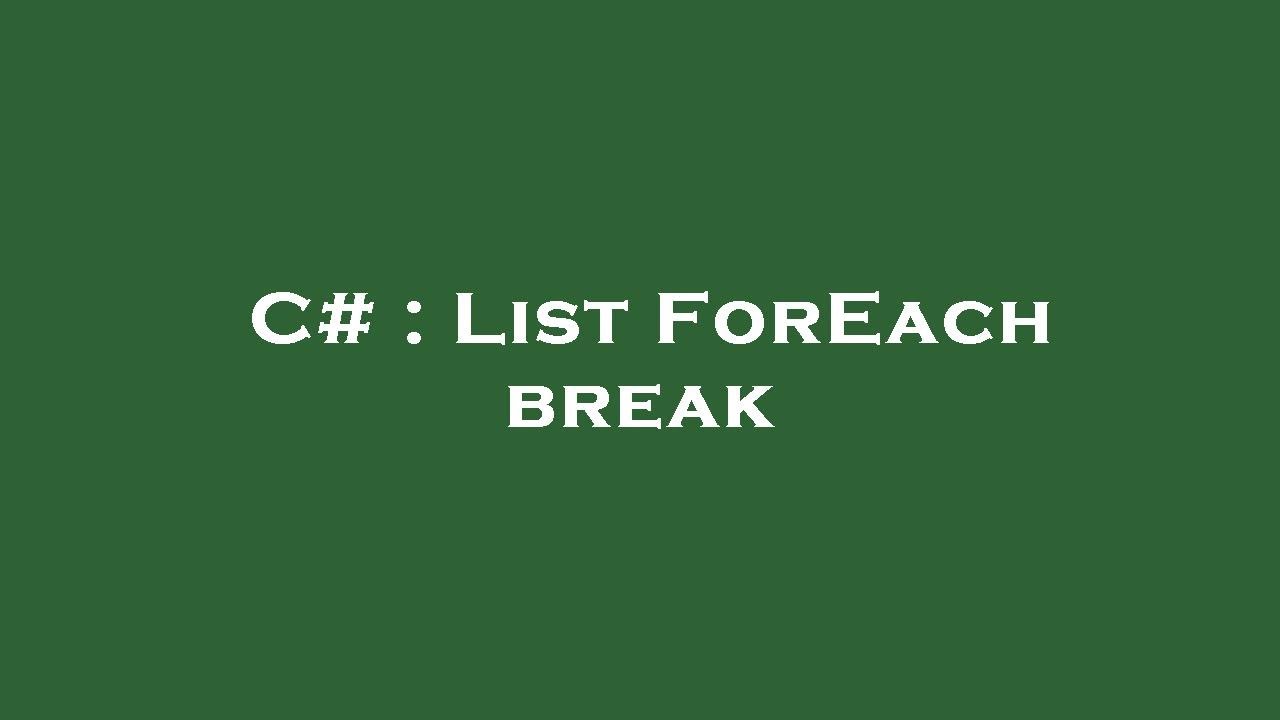