In the ever-evolving world of software development, managing dates and times effectively is crucial for the success of any application. Joda-Time has emerged as a powerful library that simplifies date and time handling in Java. Built to address the shortcomings of Java’s native Date and Calendar classes, Joda-Time provides a robust framework for developers to work with temporal data. It offers a clear and comprehensive API that enables developers to perform complex date and time manipulations with ease.
As applications become more complex, the need for precise date and time calculations has never been greater. This is where Joda-Time shines, offering a plethora of features that cater to the varying needs of developers. From managing time zones to handling daylight saving time changes, Joda-Time ensures that developers can focus on building their applications without getting bogged down by time-related issues. This guide will delve into the features of Joda-Time, how to implement it in your projects, and why it stands out in the crowded field of date and time libraries.
The popularity of Joda-Time is not just due to its extensive functionality; it also stems from its commitment to clarity and ease of use. Developers appreciate the intuitive design and the ability to create, manipulate, and format date and time objects with minimal fuss. In this article, we will explore the various components of Joda-Time, answer common questions, and provide examples that will help you integrate this library into your Java applications seamlessly.
What is Joda-Time?
Joda-Time is an open-source date and time library for Java that provides a more comprehensive and user-friendly alternative to Java’s built-in date and time handling mechanisms. It was created to simplify the complexities that come with date and time manipulations, offering a fluent API that developers can use to create and format dates, manage time zones, and execute calculations effortlessly.
Why Should Developers Use Joda-Time?
There are several compelling reasons for developers to adopt Joda-Time in their Java applications:
- Ease of Use: The API is designed for simplicity and clarity, reducing the cognitive load on developers.
- Comprehensive Functionality: It includes features for handling time zones, intervals, durations, and more.
- Immutability: Joda-Time objects are immutable, which helps avoid unexpected behavior in applications.
- Compatibility: Joda-Time is designed to work seamlessly with Java's existing date and time classes.
How Does Joda-Time Compare to Java's Built-In Date and Time Classes?
Java’s built-in Date and Calendar classes have been criticized for their cumbersome interfaces and inherent pitfalls. Joda-Time improves upon these flaws with a more straightforward and logical approach:
- More Intuitive API: Joda-Time's methods are easier to understand and use.
- Better Handling of Time Zones: Joda-Time provides accurate time zone management, which is crucial for global applications.
- Improved Performance: Joda-Time is optimized for performance, making it suitable for applications that require high efficiency.
How to Get Started with Joda-Time?
Integrating Joda-Time into your project is a straightforward process. Follow these steps to get started:
- Add Joda-Time to Your Project: Include the Joda-Time library in your project dependencies.
- Import Necessary Classes: Import the required classes from Joda-Time into your Java files.
- Create DateTime Objects: Use Joda-Time’s DateTime class to create and manipulate date and time objects.
What Are Key Features of Joda-Time?
Joda-Time boasts a variety of features that enhance its usability:
- DateTime Class: The core class for representing date and time.
- Interval Class: Represents a time interval between two moments.
- Period Class: Represents a period of time in terms of years, months, days, etc.
- Time Zone Support: Comprehensive support for different time zones and daylight saving time.
Can You Provide Examples of Joda-Time in Action?
Certainly! Below are a few examples that demonstrate the power of Joda-Time:
import org.joda.time.DateTime; import org.joda.time.Interval; public class JodaTimeExample { public static void main(String[] args) { DateTime now = new DateTime(); System.out.println("Current DateTime: " + now); Interval interval = new Interval(now.minusDays(5), now); System.out.println("Interval Length: " + interval.toDuration().toStandardHours().getHours() + " hours"); } }
What Are the Common Pitfalls to Avoid When Using Joda-Time?
While Joda-Time is a powerful tool, there are some common pitfalls to be aware of:
- Not Understanding Immutability: Remember that Joda-Time objects are immutable; any modification creates a new object.
- Ignoring Time Zones: Always be mindful of time zone differences when performing date calculations.
- Overcomplicating Simple Tasks: Use Joda-Time’s features appropriately to avoid unnecessary complexity.
What Is the Future of Joda-Time?
As the Java ecosystem continues to evolve, so does Joda-Time. While the Java 8 Date and Time API has introduced new features, Joda-Time remains relevant due to its ease of use and comprehensive functionality. Developers can expect continued support and updates to the library, ensuring that it remains a valuable tool for date and time management in Java applications.
Conclusion: Is Joda-Time Right for Your Project?
In conclusion, Joda-Time offers a powerful, user-friendly solution for managing dates and times in Java applications. Its intuitive API, comprehensive feature set, and strong community support make it an excellent choice for developers seeking to simplify their date and time handling. Whether you are building a small application or a large enterprise system, Joda-Time can help you navigate the complexities of temporal data with ease and precision. So, is Joda-Time the right choice for your project? The answer is a resounding yes for developers looking for clarity and efficiency in their date and time management.
Article Recommendations
- Elements Compounds And Mixtures Answer Key
- Gen Tullos
- Reflex Compound Bow
- Robin Sharma Wife
- Frost Line In Alaska
- Mexican Pot Luck
- Water Dam For House
- Freddie Prinze Jr Jessica Biel
- Sarah Lahbati Starstruck
- Horny In Sign Language

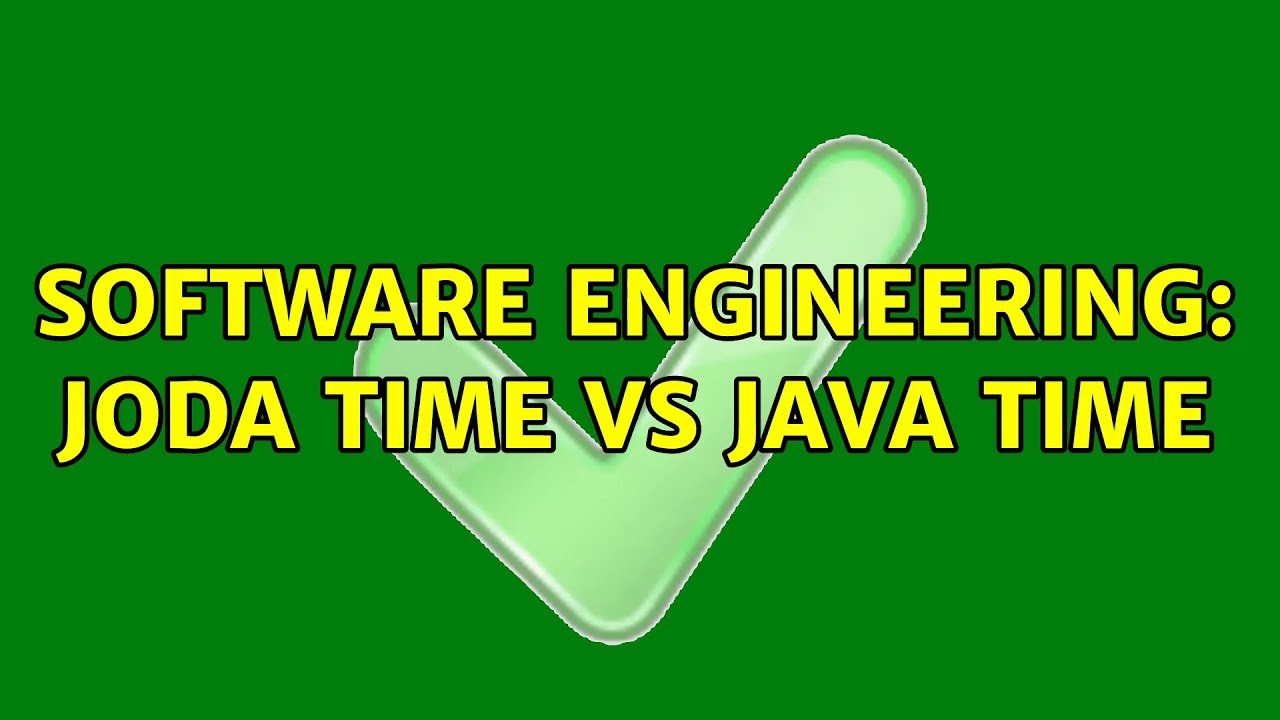
